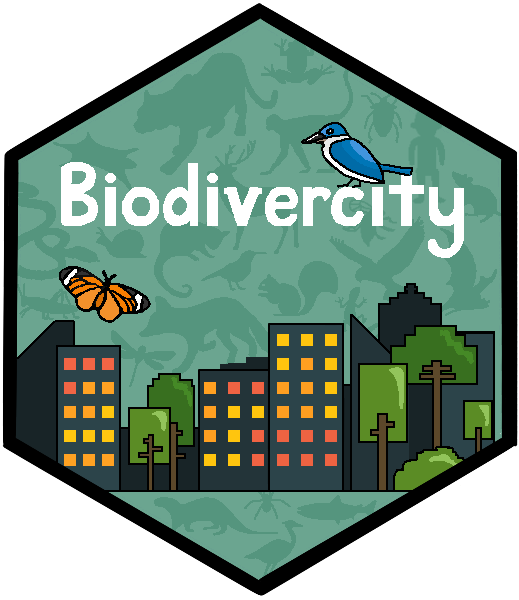
Simulate multiple scenarios with animal surveys randomly excluded
Source:R/exclude_simulator.R
exclude_simulator.Rd
Randomly exclude either animal surveys or sampling points (and all their associated surveys) from a species community matrix. Each column of the community matrix should represent a unique species, while each row represents a single unique survey.
Usage
exclude_simulator(
community_matrix,
survey_ref,
exclude_level = "survey",
exclude_num,
specify_areas = NULL,
specify_points = NULL,
specify_periods = NULL,
specify_cycles = NULL,
area_ignore = NULL,
period_ignore = NULL,
cycle_ignore = NULL,
survey_id = "survey_id",
point_id = "point_id",
area = "area",
period = "period",
cycle = "cycle",
rep = 100
)
Arguments
- community_matrix
Dataframe of the species community matrix (one survey per row). It should a column containing the unique IDs for the animal surveys conducted (e.g.
survey_id
).- survey_ref
Dataframe containing information of the surveys conducted (e.g.
survey_id
,point_id
,period
,area
,cycle
). Values in the columnsurvey_id
should correspond to those incommunity_matrix
.- exclude_level
Whether to exclude based on
"survey"
or"point"
. Defaults to"survey"
.- exclude_num
Number of surveys/points to exclude (remove) from the full dataset
community_matrix
. Value should be a single integer.- specify_areas
Specify the
area
(s) to randomly exclude surveys from (character string or vector). Defaults toNULL
, which excludes surveys randomly across all areas.- specify_points
Specify the
point_id
(s) of sampling points to randomly exclude surveys from (character string or vector). Defaults toNULL
, which excludes surveys randomly across all points.- specify_periods
Specify the survey
period
(s) to exclude surveys from (character string or vector). Defaults toNULL
, which excludes surveys randomly across all periods.- specify_cycles
Specify the survey
cycle
(s) to exclude surveys from (character string or vector). Defaults toNULL
, which excludes surveys randomly across all cycles.- area_ignore
Ignore specified
area
(s) from the simulation and output (character string or vector). Defaults toNULL
, which includes all areas in the simulation.- period_ignore
Ignore specified
period
from the simulation and output (character string or vector). Defaults toNULL
, which includes all periods in the simulation.- cycle_ignore
Ignore specified
cycles
(s) from the simulation and output (character string or vector). Defaults toNULL
, which includes all cycles in the simulation.- survey_id
Column name of the unique identifier for each survey in
community_matrix
andsurvey_ref
. Defaults tosurvey_id
.- point_id
Column name of the unique identifier for each sampling point in
survey_ref
. Defaults tosurvey_id
.- area
Column name of the area specified in
survey_ref
. Defaults toarea
.- period
Column name of the survey period specified in
survey_ref
. Defaults toperiod
.- cycle
Column name of the survey cycle specified in
survey_ref
. Defaults tocycle
.- rep
Specify number of repetitions (scenarios) to simulate. Defaults to
100
.
Value
A dataframe containing the results of each iteration (column).
The count of sampling points with each species (row) is shown. The column full
shows the breakdown of counts within the original dataset.
Examples
data(animal_observations)
data(animal_surveys)
# filter animal observations to taxon of interest
birds <- filter_observations(observations = animal_observations,
survey_ref = animal_surveys,
specify_taxon = "Aves")
# convert animal observations to community matrix
birds <- as.data.frame.matrix(xtabs(abundance ~ survey_id + species, data = birds))
birds <- cbind(survey_id = rownames(birds), birds) # convert rownames to col
# run function
set.seed(123)
exclude_simulator(birds, animal_surveys,
exclude_num = 15, exclude_level = "survey", # exclude 15 surveys
specify_cycles = 5, # exclude those in cycle 5 only
period_ignore = "2", # remove all data from period 2
rep = 10) # 10 scenarios only
#> # A tibble: 138 × 12
#> species full iter1 iter2 iter3 iter4 iter5 iter6 iter7 iter8 iter9 iter10
#> <chr> <int> <int> <int> <int> <int> <int> <int> <int> <int> <int> <int>
#> 1 Accipiter… 1 1 1 1 1 1 1 1 1 1 1
#> 2 Accipitri… 1 1 1 1 1 1 1 1 1 1 1
#> 3 Acridothe… 157 157 157 157 157 157 157 157 157 157 157
#> 4 Acridothe… 52 51 51 52 52 52 52 52 52 52 51
#> 5 Acrocepha… 1 1 1 1 1 1 1 1 1 1 1
#> 6 Acrocepha… 1 1 1 1 1 1 1 1 1 1 1
#> 7 Actitis h… 4 4 4 4 4 4 4 4 4 4 4
#> 8 Aegithina… 68 67 67 68 68 67 67 66 67 67 67
#> 9 Aerodramu… 1 1 1 1 1 1 1 1 1 1 1
#> 10 Aerodramu… 1 1 1 1 1 1 1 1 1 1 1
#> # … with 128 more rows